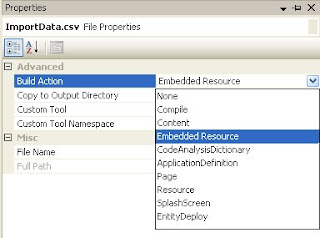
While I was writing the unit test for one of the scenario, where I need to get the data from a csv file from the specified path, for running my unit test. Then comes, the question - How can i add the file to the solution and the get the necessary data from the file and what are the ways? There are two options
- First mark the csv file as an embedded resource in the solution, and have a little utility to copy the file to your temp folder and get the path from there.
- Mark the csv file as an embedded resource in the solution, and then stream the file straight from the assembly.
The second option is probably preferred because you don't pick up the bottle neck of touching the disk, but unfortunately, my test method accepts the path of the file name, so i have to write a utility to read the file from the assembly, and copy it to the temp folder. Here are the steps to work it out.
To add an file as embedded resource to the project, follow the steps below.
Since the test file will be available with us, just add the file to the project, by choosing Add Existing Item menu in the right click option of the project.
After adding the project, go to the properties of the file added, In the Build Action selection embedded resource, and rebuild the project, that's it your files has been added to the project as an embedded resource.
So now how to read the embedded resource, and create a temporary file.
With the help of assembly.GetMainfestResourceStream(resourceName), you can get the resource stream and the Path.GetTempFileName(), creates a uniquely named, zero byte temporary file on the disk, and returns the full path of that file, and now write the stream to the specified path.
Below is the sample which reads the embedded resource and creates a temp file in the temporary folder.
private const string SampleFile = "TestProject.CRM.ImportData.csv";
public static string UnpackManifestResource(string resourceName, string extenstion, Assembly assemblyName)
{
// Extract the specified resource as a stream
Assembly assembly = assemblyName;
if(assemblyName == null)
assembly = Assembly.GetExecutingAssembly();
string targetPath = Path.GetTempFileName();
if (!string.IsNullOrEmpty(extenstion))
targetPath = Path.ChangeExtension(targetPath, extenstion);
using (Stream resourceStream = assembly.GetManifestResourceStream(resourceName))
{
if (resourceStream != null)
{
var readBytes = new byte[resourceStream.Length];
resourceStream.Read(readBytes, 0, (int)resourceStream.Length);
using (var outputStream = new FileStream(targetPath, FileMode.Create))
{
outputStream.Write(readBytes, 0, readBytes.Length);
}
}
}
return targetPath;
}
No comments:
Post a Comment